Understanding the call stack is essential for flow control and organization of code execution in various types of programs. It is important to note that each programming language has its implementation of the call stack, and therefore there may be differences in how it is handled in each.
How does Call Stack in JavaScript works?
JavaScript is a programming or scripting language that allows you to implement complex functions on web pages. The JavaScript engine only has one call stack. Therefore, it can only do one thing at a time. When you run a script, the JavaScript engine runs the code from top to bottom, line by line, synchronously.
This programming language uses the call stack to keep track of various function calls. It's like a real stack in data structures, where data can be pushed and popped, following the Last In, First Out ( LIFO ) principle to temporarily store and manage the function invocation ( call ).
This means the last function pushed to the stack is the first to appear when the function returns. The script will stop when the call stack is empty.
The call stack is where our code is executed at its most basic level, using the execution context.
To do this, the JavaScript engine uses an event loop, which checks if there are any function calls on the call stack, takes the function call, and executes it.

JavaScript call stack example
function add(a, b) {
return a + b;
}
function average(a, b) {
return add(a, b) / 2;
}
let x = average(10, 20);
The steps and illustrations below explain the call stack of the function above.
When the JavaScript engine executes this script, it places the global execution context (indicated by main() global() function on the call stack.

The global execution context enters the creation phase and proceeds to the execution phase.
The JavaScript engine executes the call to the average(10, 20) function and creates a function execution context for the average() function, and places it on top of the call stack:
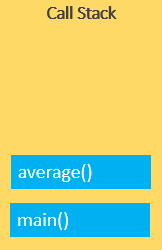
The JavaScript engine starts executing average() from because the average() function is at the top of the call stack.
The average() function calls add() to the function. At this point, the JavaScript engine creates another function execution context for the add() function and places it on top of the call stack:

The JavaScript engine executes the add() function and pops it off the call stack:
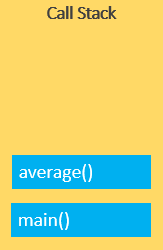
At this point, the average() function is on top of the call stack, and the JavaScript engine executes and pops it off the call stack.
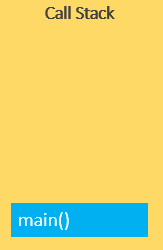
Now the call stack is empty, so the script stops executing:
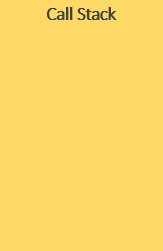
The following image illustrates the general state of the call stack in all steps:

Stack Overflow and RangeError
It is also important to note that the Call Stack is a data structure limited in size. If your application has many nested or recursive functions, it can quickly consume space in the Call Stack and cause errors. If the number of execution contexts exceeds the size of the stack, a stack overflow error will occur.
For example, when you execute a recursive function that does not have an exit condition, the JavaScript engine will generate a stack overflow error:
function fn() {
fn();
}
fn(); // stack overflow
What causes these errors in the call stack?
- Problems in handling recursion.
- If you have a function that calls itself multiple times, multiple stack frames will be generated in the Call Stack, which can cause a stack overflow and cause your application to crash.
- Operations out of range.
How to solve the errors?
The Stack shows us the execution order of our program, which is very useful when solving errors. To resolve the error, determine the cause and specify a condition that will exit the recursion. If you encounter this error when calling recursive functions, you must ensure that the function has a base case defined to terminate recursive calls.
If this error occurs due to excessive calls to functions or variables, these should be reduced to the maximum. Any out-of-range operations should also be checked and avoided. These issues can be inspected using the browser console and developer tools.
function myFunc(i) {
if (i >= 5) {
return;
}
myFunc(i+1);
}
myFunc(1);
The above code avoids the error as the recursive calls terminate when the base case is true.
Conclusion
In conclusion, the call stack is a fundamental programming tool used to monitor the execution of various processes or subroutines in a program.
You can create more efficient and functional applications With good knowledge and management of the call stack.
This is why it is essential to understand how it works and how it can affect application performance. Understanding the hierarchy of functions and the order of execution in the JavaScript engine is key to optimizing this tool's proper and efficient use.
Frequently asqued questions
What is the difference between stack and call stack?
The stack is a memory structure that stores temporary data like variables and function calls in a Last-In, First-Out (LIFO) order while the call stack specifically manages function calls in a program, tracking each function's state so that when a function finishes, control returns to the previous function.
What is the difference between call stack and queue?
A call stack is a LIFO (Last-In, First-Out) structure that manages function calls, with the last function called being the first to finish while a queue is a FIFO (First-In, First-Out) structure where the first item added is the first to be processed, commonly used in tasks like scheduling and message handling.