How does blockchain work?
A blockchain works by maintaining a digital ledger of transactions across a network of computers. Each computer on the network, called a node, has a copy of the ledger, and the nodes work together to ensure that the ledger is accurate and up-to-date.
- Transactions: A transaction is initiated when one person sends digital assets (e.g., cryptocurrency, digital certificates) to another person. The transaction includes information such as the sender and recipient and the number of digital assets being transferred.
- Verification: The transaction is verified by the nodes on the network using a consensus mechanism. The most common consensus mechanism is called "proof of work," which involves nodes solving a complex mathematical problem to validate the transaction.
- Block creation: Once a transaction is verified, it is added to a block, along with other verified transactions. Each block contains a cryptographic hash of the previous block, a timestamp, and transaction data.
- Chain: The blocks are linked chronologically, forming a chain of blocks, hence the name "blockchain". Each block in the chain contains a copy of the previous block's hash, which creates a link between the blocks and ensures that the data in the blocks cannot be altered.
- Distributed ledger: Each node on the network has a copy of the blockchain, which is updated in real-time as new blocks are added. This creates a distributed ledger, where all nodes have the same information and no central control point.
- Smart contract: Smart contracts are self-executing contracts with the terms of the agreement written directly into lines of code, it's a program that runs on the blockchain and can be used to automate various types of transactions.
One of the key benefits of a blockchain is that it is a decentralized and distributed system, which makes it more resistant to tampering and hacking. Additionally, using cryptography ensures that transactions are secure, and the consensus mechanism ensures that the ledger is accurate and up-to-date.
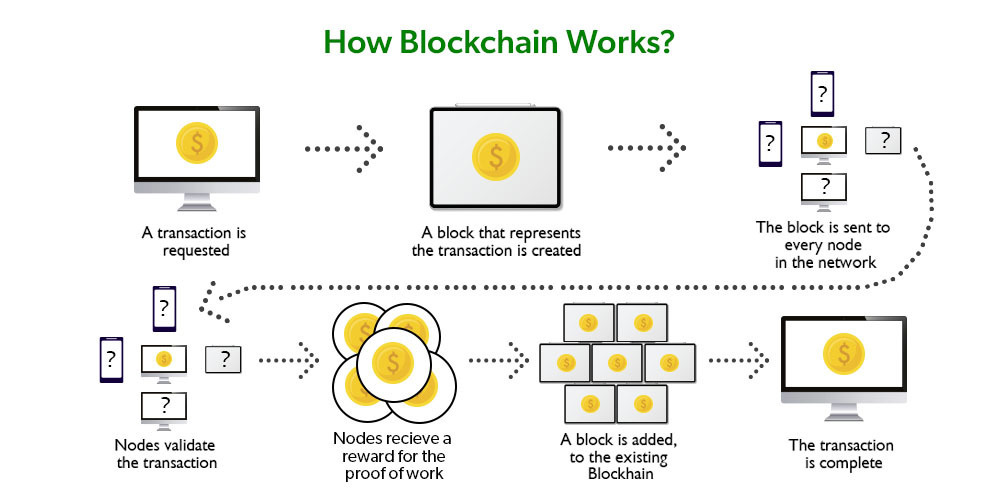
How to implement a Blockchain?
A blockchain is implemented through a combination of software and network protocols. The software is responsible for maintaining the ledger and the network protocols are responsible for ensuring the security and integrity of the data on the ledger.
Here are the main components of Blockchain implementation
Node software: Each computer on the network runs a copy of the node software, which is responsible for maintaining the ledger. The node software includes the blockchain protocol, responsible for validating new transactions and adding them to the ledger.
Network protocol: The network protocol is responsible for ensuring that all nodes on the network have a copy of the same ledger and that new transactions are broadcast to all nodes. This is typically done through a peer-to-peer network, where nodes can connect and share data.
Consensus mechanism: A consensus mechanism is used to validate new transactions to ensure that all nodes have the same copy of the ledger. The most popular consensus mechanism is "proof of work," which involves nodes solving a complex mathematical problem to validate a new block of transactions
Cryptography: Cryptography is used to secure the data on the blockchain by creating a digital signature for each transaction. This signature is based on the private key of the sender, and it is verified by the public key of the recipient.
Smart Contract: Smart contracts are self-executing contracts with the terms of the agreement written directly into lines of code; it's a program that runs on the blockchain and can automate various types of transactions.
To implement a blockchain, the first step is to choose a consensus mechanism and design the network protocol. Then, the node software and smart contracts can be developed, and the network can be launched. Ongoing maintenance and updates are also necessary to ensure the security and scalability of the blockchain.
Can we implement a blockchain with JavaScript?
Yes, it is possible to implement a blockchain with JavaScript. JavaScript is a widely used programming language with a large developer community, making it a good choice for implementing a blockchain.
Several JavaScript libraries and frameworks are available for building a blockchain, such as:
- Node.js: This is a JavaScript runtime that allows for the development of server-side applications. Node.js is often used for building decentralized applications on the Ethereum blockchain.
- Web3.js: This is a JavaScript library that allows for interaction with the Ethereum blockchain. It can be used to create and deploy smart contracts and to send and receive transactions on the Ethereum network.
- Crypto-js: It is a JavaScript library that provides cryptographic functions such as hashing, encryption, and digital signature. It can be used to secure data on the blockchain.
- Hyperledger Fabric: it is an open-source blockchain framework and one of the Hyperledger projects hosted by the Linux Foundation. It uses Chaincode, which is written in JavaScript, to implement smart contracts.
- IOTA: it's a distributed ledger technology that uses a new data structure called Tangle; it's written in JavaScript and can be used for creating distributed systems.
Building a blockchain with JavaScript requires a solid understanding of the underlying concepts and technologies, including distributed systems, cryptography, and consensus mechanisms.
It's important to note that building a blockchain from scratch can be challenging and requires a lot of work; it's recommended to use an existing blockchain platform or framework and build on top of it.
How to implement a blockchain with JavaScript?
Here is a simple example of how a blockchain can be implemented in JavaScript. This example uses a basic data structure to represent the blockchain and includes methods for adding new blocks and validating the blockchain.

This example is a fundamental implementation of blockchain, it doesn't include some important aspects of a blockchain like a consensus mechanism, security, and scalability. It's not recommended to use this example in a production environment; it's just to illustrate the basic concepts of blockchain.
It's important to note that building a blockchain from scratch is a complex task and requires a lot of work, and using existing blockchain platforms or frameworks to build on top of them is recommended.
The last piece of code is an example of how a blockchain can be implemented in JavaScript. It uses a basic data structure to represent the blockchain, including methods for adding and validating new blocks.
The example has two main classes: "Block" and "Blockchain".
The "Block" class represents a block in the blockchain, and it has four properties:
- "index": A unique number that identifies the block in the chain.
- "timestamp": The time at which the block was created.
- "data": The data that is stored in the block. This can be any type of data, but it is a simple string in this example.
- "previousHash": A reference to the hash of the previous block in the chain.
- "hash": A cryptographic hash of the block.
The "Block" class also has a method called "calculateHash()" which creates a SHA256 hash of the block data. This hash ensures the integrity of the data stored in the block.
The "Blockchain" class represents the blockchain, which has an array called "chain" that stores the blocks. It has four main methods:
- "createGenesisBlock()": This method creates the first block in the blockchain, called the Genesis block. It sets the index to 0, timestamp to the current time, data to "Genesis Block" and the previousHash to "0".
- "getLatestBlock()": This method returns the last block in the blockchain.
- "addBlock(newBlock)": This method adds a new block to the blockchain. It sets the previousHash property of the new block to the hash of the current last block and then calculates the new block's hash using the "calculateHash()" method.
- "isChainValid()": This method checks if the blockchain is valid. It iterates through all the blocks in the blockchain and compares the calculated hash of each block with the stored hash. If the calculated hash is different from the stored hash, or if the previousHash of a block does not match the hash of the previous block, the method returns false, indicating that the blockchain is not valid.
This example is a fundamental blockchain implementation; it doesn't include some important aspects, like a consensus mechanism, security, and scalability. It's not recommended to use this example in a production environment; it's just to illustrate the basic concepts of blockchain.
If you are interested in implementing a blockchain with JavaScript just for the sake of learning how it works i recommend this series on Youtube that takes you through the process step by step.
Frequently Asqued Questions
Is JavaScript necessary for blockchain?
JavaScript is not strictly necessary for blockchain but is widely used for developing blockchain applications, especially for web-based interfaces and smart contracts integration.
Is Python or JavaScript better for blockchain?
Python is better for blockchain development when focusing on data-heavy applications and quick prototyping. JavaScript excels in building web-based blockchain interfaces and decentralized applications (dApps). The choice depends on the project's needs.
Is blockchain hard to code?
Blockchain can be challenging to code due to its complexity, requiring knowledge of cryptography, distributed systems, and smart contracts. However, tools and frameworks have made it easier for developers to build blockchain applications.