Along with this, some sophisticated implementations of JavaScript allow for developing server-side functionality via NodeJS, which enables developers to program javascript-based web servers.
Client-side, at its core, is all about managing, changing, moving, and updating the DOM (Document Object Model), a representation of the web page which is rendered, to present several different scenarios and client-side functionality like displaying dynamic content, moving images, adapting functionality for updating images, and even more, all of this related and interconnected to create a seamless experience and the UX (User Experience).
Several framework implementations have been developed for better and faster creation of web applications, such as React, VueJS, and Angular.
Along with these, several UI frameworks have been set too, which implement ready-to-use UI styles and look n’ feels that improve the user experience.
Server-side JavaScript is basic and implements the barebones of Object Oriented programming language functionality through something called NodeJS.
However, JavaScript is often used with several other implementations through package managers, such as NPM or Yarn, to expand and enforce several other functionalities.
These two are the most common and allow you to implement external functionality with simple installation commands (for example, npm install axios).
This means that JavaScript can alter the appearance of the webpage (DOM) in the browser. Similarly, Node.js JavaScript on the server can respond to custom requests sent by browser code and implement server-side functionality.
However, both environments can execute the same base code (JavaScript) and can frequently be interchangeable when debugging and developing brand-new functionality that requires no external dependencies (for example, a simple algorithm that calculates some values by executing some basic algebraic operations) or that use base libraries and functionality built for different environments (such is the case of Math library).
Scripting for fun and profit
JavaScript is called -Script because it implements a programming language based on the Script paradigm, where scripts (which could be defined as a sequence of instructions or commands for a computer to execute’) are executed in an orderly manner by the JavaScript engine turn.
An essential distinction between scripting and other paradigms is that a script doesn’t necessarily need to be compiled.
It just needs to be read and executed by the machine (i.e., it doesn’t need to be transformed in any meaningful manner, differently from some other languages, for example, Java, which requires to transpile the code into Java Bytecode, depending on the executing environment). It’s just read and executed accordingly.
This makes JavaScript an easy-to-run, easy-to-practice language, beneficial due to its immediate feedback (it can present a short learning curve for starters, which, with the right approach, can help you leverage into meaningful tasks such as updating the pages you visit however you want, extracting meaningful data (ex. Emails, phone numbers, and basically, anything you want)), automating actions on the browser and server, and more.
And one of the best things about JavaScript is that you can execute it on any browser you’re working on. For example, you can execute Ctrl + Shift + J on Google Chrome, which will pop up the developer's tools.
You can leverage this by clicking on the Console tab on top of it

And you can execute your first “Hello World” by writing:
console.log("Hello World!!");
And hitting enter.

This will allow you to execute your first JavaScript command!! If undoubtedly it can look quite simple, it’s the first step to developing more complex, meaningful scripts that will make your life easier.
One language to rule them all: Why JS?
Now, the question might come to mind: why JavaScript? What does it do that other languages don't? Why should you pay attention to it more frequently than other languages?
The answer is: you don’t have to.
JavaScript is a complementary language that can (and is often encouraged to) be used in conjunction with other languages, such as server-side languages (Java, Python, Go, Etc) that provide the required functionality for websites to contain backend functionality.
However, the ease of learning and the minor breach between server-side and client-side JavaScript makes it ideal for full stack development, where you won’t have to worry about different languages on different syntax; instead, you can focus on the solutions instead of learning the ins and outs.
TypeScript and friends: an introduction to typed JS.
Despite JavaScript’s ease of learning and application, we quickly realize that we need to provide some sort of type annotations for complex programs and complex systems.
Often we need to provide some way to structure our code more efficiently and in a maintainable manner. Despite JavaScript's flexibility (one of its features), we also need some typing.
This problem is not found in other languages, such as Java, where the typing is static. The errors can be caught at build time and provide specific non-dynamic data structures and data types that allow for structured code.
To implement the same in javascript, we need to use typing, which allows us to set definite structures to the code. This, in turn, requires a compilation phase where the code written is analyzed, and any issues that might arise as the result of bad coding practices or illegal statements are detected before the code can be ready for use.
This is done through an intermediary language called TypeScript.
Typescript allows for static checking of values and can catch errors before the execution of the code. It is a typed superset of javascript, and you can implement JavaScript syntax directly on typescript.
The main difference between typescript and JavaScript is that typescript is a superset of JavaScript; meanwhile, JavaScript is the base language, so if you're not sure what to learn first, keep in mind they are both intertwined, and by learning JavaScript first, you can eventually implement typescript as well without too many issues.
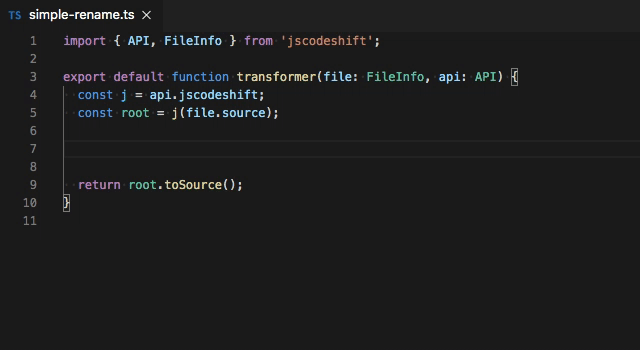
Practical study methodologies for learning JavaScript
When learning to code in JavaScript, you need to remember that it can be a lonely journey where you need to keep a long time in front of your computer and understand concepts intimately.
This can often lead to people stopping coding and giving up learning to code entirely.
However, it doesn't have to be a different way, and you can implement several approaches to solve these issues and avoid falling into the trap of your own making.
Project Based Approach
Programming is a tool that you can use to improve your life and expand on the things you can do. For example, you can use programming to automate actions on a website you like; you can use programming to solve issues and make calculations in an automated manner or simply make something that didn't exist before.
Based on this fact, you can implement several projects into your life and learn to program simultaneously.
Your imagination is the limit, and different programs and libraries can ease the development speed of whatever project you intend to create.
The Feynman Technique
This is based on the "if you want to understand something, try to explain it simply." The angle of this technique is to explain something in simple terms. You take a concept and try to explain it simply, then you review what you wrote and check for inconsistencies and errors.
This way, you can fix issues in your reasoning and improve your understanding of that concept.
Resume and abstracts
Another technique you can use (that you probably used in school as well) is to make resumes and abstracts of the material you read and study, and that way, you can have a better concept on your own of whatever you're trying to learn.
Community
You can learn a lot by joining a particular community of developers and contacting other like-minded people like yourself. Reddit and Facebook groups are places where developers often hang out and interchange questions and answers. The most crucial part is not to isolate yourself while learning to program.
Where to now?...
There are several resources on the web which can help in learning JavaScript. These are some of the best places on the internet to continue learning JavaScript.
- https://www.w3schools.com/ This is an old-school learning platform where you can learn Web concepts and web development in general.
- https://www.codecademy.com/ is one of the best places to learn to program, where the website is tailored to your particular profile and will lead you through several lessons that will teach you programming in a fun interactive manner.
- https://www.youtube.com/ there are several channels and tutorials on how to learn JavaScript, and the best part is that they are entirely free, and you can access them anywhere.
We’ll also continue this series of JavaScript tutorials and delve deeper into what JavaScript is and how we can use it for real-life scenarios. The methodologies to work with it that will ease up the development of your projects.